Working with ASP.Net controls to Insert, Update and Delete with SQL Server Database - No Coding
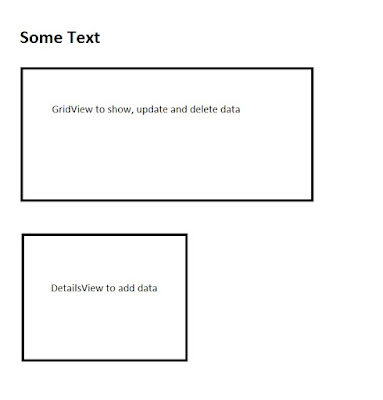
Working with ASP.Net controls to Insert, Update and Delete with SQL Server Database - No Coding This blog will teach you how to create a simple application using ASP.Net controls and MS SQL database. We are going to do this without any back end C# codeings. :) Following shows the page that we are going to create. It has function to: 1. Show some data (I use Employee data) 2. Manage those data (Insert, Update, Delete) Step 1 Go to Visual Studio. Create a "ASP.NET Empty Web Application". Step 2 Create a new aspx page. I'll create a page called home.aspx. Step 3 To show, update and delete data we will use - ASP GridView To insert new data we use - ASP DetailsView To bind the data to these controls we use - SqlDataSource Drag and drop following ASP controls from the Visual Studio toolbox to the home.aspx. Switch to the design mode and drag and drop those controllers as you want. On the GridView and the DetailsView, set the SqlDataSource