Angular 4 Notes (Part 5) - Navigation using Angular Routing
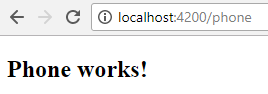
Angular Routing allows navigation between different views. Learn more on: https://angular.io/guide/router Lets try this with an example. 1. Create another component inside components folder. I named it as phone. 2. Go to phone.component.html and change the html a bit. < h2 > Phone works! </ h2 > 3. Go to app.module.ts and import router module import { RouterModule , Routes } from '@angular/router' ; 4. Import router module inside imports RouterModule . forRoot ( appRoutes ) 5. Create the routs in app.module.ts Each route will have an object. const appRoutes : Routes = [ { path: '' , component: UserComponent }, { path: 'phone' , component: PhoneComponent }, ]; 6. Change app.component.html and change its html to: < router-outlet ></ router-outlet > 7. Save and go to your browser and type: http://localhost:4200/phone then it will show your phone component. 8. Show navigation links.